There are a lot of steps you need to take before going to an interview. Even when you complete them all, applying for your first programming job can be an intimidating process, especially when you do not have any professional experience to back up your technical knowledge. A good way to showcase your practical competence is to set up a GitHub profile with all the projects you have completed so far. In addition to assessing your coding skills, an interview serves as an opportunity for the recruiter to get to know you better and determine whether or not you would fit well into the company culture.
Although every organization has a different interviewing policy, the process usually includes the following steps:
- Initial Conversation with a hiring manager;
- Technical Interview with a software developer;
- Coding Challenges.
There are three general groups of questions corresponding to each stage of the interview process – personal, technical, and practical. To give you a better idea of what to expect, we made a list of the most common ones and included hints on how to best answer them.
Personal Questions
In most cases, you will first speak with a hiring manager, who will ask you open-ended questions common to most job interviews, irrespective of the industry:
- Tell me about yourself.
Hint: You do not have to go into too many personal details. After all, you are there on a business matter. Share what sparked your interest in programming and what you expect to gain from the role you are applying for. Explain why you are a good fit for this position, and which of your skills and abilities would benefit the company and make you stand out as a candidate.
- Where do you see yourself in five (or ten) years time?
Hint: The purpose of this question is to find out if you plan in the long term and whether your goals align with the position you are applying for.
- How did you hear about our company?
Hint: Be prepared with specific information about the company. Look for information in forums, blogs, on the company’s Web site, etc. Research their goals and mission and the position you are applying for and mention how they align with your future plans.
- What are your greatest strengths and weaknesses?
Hint: Mention actual personal qualities that are relevant to the current position. Give context, details, and an example from your own experience or a potential situation in which you would use these strengths to get through a challenge.
When talking about your weaknesses you want to give a well-thought answer that does not sound too rehearsed. The best way is to give an example of a small shortcoming you are working on overcoming. Share what you have learnt from the process and how you will use the experience in your future work.
- What are your hobbies and interests?
Hint: To dig deeper into your character, the hiring manager might ask you about your interests outside of work. Mention hobbies that you are really into, but make sure to clarify that they will not affect your dedication to the job. Sharing your interests outside of work is a genuine way to show your personality and connect with the interviewer.
Technical Questions
The interview will continue with a test of your technical knowledge in the form of some behavioral and theoretical questions. At this stage, you are likely to have a software engineer who will ask you progressively harder theoretical questions. Although the technical part of an interview is highly dependent on the job requirements, there are some general questions at the start, like:
- Why did you choose this programming language, and what interests you about it?
Hint: Express your understanding of the technology you chose. Describe its features, advantages, and disadvantages, as well as the applications you can develop with it. To show that you have at least a basic understanding of other languages, you can also draw comparisons with some of them.
- Describe the steps you take to develop a program, from getting the assignment or an initial idea to its final execution.
Hint: Explain the actual sequence of steps you take when developing a program. Interviewers are particularly interested in your project planning process, what coding techniques you use, and whether you follow the best practices that guarantee high-quality and readable code.
- How do you debug your code and approach problems?
Hint: Since troubleshooting and debugging are crucial components of a software engineer’s work, it is important to describe how you handle issues. This will demonstrate your level of logical and algorithmic thinking and problem-solving skills.
The more in-depth questions are usually theoretical, so it is entirely up to you to be prepared beforehand. This is a sample list of technical questions for four of the most popular programming languages for web development, as well as a separate section about Object Oriented Programming (OOP).
Java
- What are the main features of Java?
- Why is Java platform-independent?
- What is the difference between the JDK, JRE, and JVM?
- How do Heap and Stack memory differ?
- Explain the access modifiers used in Java.
- What is the role of the garbage collector?
- What is Final word in Java used for?
- What is the difference between Stack and Queue?
C#
- What are the main features of C#?
- How is C# compiled?
- What is the difference between managed and unmanaged code?
- Explain the different types of classes.
- Explain the access modifiers used in C#.
- What are boxing and unboxing in C#?
- What are the main differences between Array and ArrayList in C#?
- What is the difference between throw exception and throw clause?
Python
- What are the main features of Python?
- What is the difference between list and tuples?
- What are the built-in data types?
- What is a namespace?
- What is PEP?
- How is Python interpreted?
- What are local and global variables?
JavaScript
- What are the main features of JavaScript?
- What are the data types in JS?
- What is the difference between ViewState and SessionState?
- What are JavaScript Cookies?
- What is the difference between pop() and shift() method?
- What are the disadvantages of using innerHTML?
- What are the different types of errors in JavaScript?
- What is the Strict Mode in JavaScript, and how can it be enabled?
OOP
- What are the principles of OOP?
- What are the features of OOP?
- What is the Singleton Class and how to implement it?
- What is inheritance?
- What is the difference between a class and an object?
- Explain superclass and subclass.
- What is the difference between method overloading and method overriding?
These questions can be asked specifically about your projects, in case you have any uploaded on your GitHub profile. The interviewer might be interested in discussing the technologies and practices that you used to develop them.
Coding Challenges
In addition to the theoretical questions, you might be given a problem to solve. It can be a take-home assignment or a coding challenge to do live during the interview. Make sure you are familiar with the syntax of the programming language required for the position you are applying for, because a coding challenge may require you to debug a block of code or determine what its result would be. It is possible that the code is intentionally made confusing in order to gauge how you would respond when faced with a challenge or in a stressful situation. In this case, the most important things are to stay calm, fight off any anxiety, and try to find a logical and well-structured solution to the problem. Demonstrating genuine enthusiasm for coding, algorithmic thinking, and a desire to learn is sometimes more important than coming up with the right answer.
Listed below are some basic junior coding challenges and hints on how to resolve them:
- Factorial
The factorial of a positive integer is the sum of the multiplication of all the integers smaller than that positive integer. For example:
4! = 4*3*2*1 = 24
5! = 5*4*3*2*1 = 120
Task: Write a program that takes a positive integer as an input and calculates the factorial of that number.
Hint: To solve this problem, use either a loop or recursion.
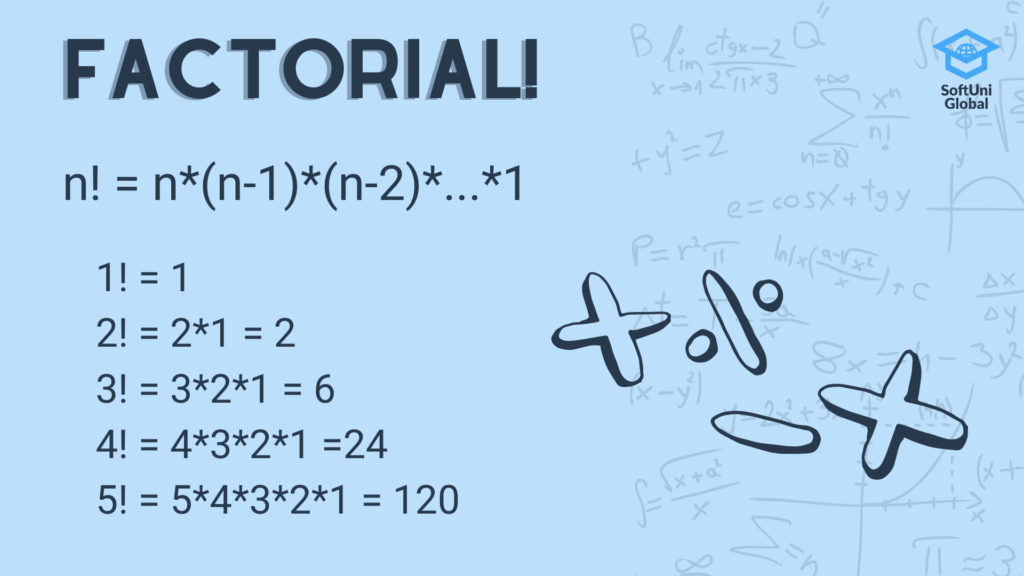
- Palindrome
A palindrome is a sequence of characters which reads the same backward as forward. For example, “radar”, “rotator”, and even longer phrases like “pull up if I pull up”.
Task: Write a program that takes a string as an input and returns if it is a palindrome.
Hint: You can:
- Check if the input is a string;
- Turn it to lower or uppercase;
- Reverse it (using a method or a loop);
- Check if the reversed string is equal to the input string;
- Return the result.
- FizzBuzz
Task: Write a program that counts the numbers from 1 to 100 on a new line.
If the number is divisible by 3, print “Fizz”.
If the number is divisible by 5, print “Buzz”.
If the number is divisible by both 3 and 5, print “FizzBuzz”.
Otherwise, print the number.
Hint: Make a loop that iterates over the numbers, and for every number, check the given conditions using the remainder operator. Be careful with the order of conditions because it can be crucial to the end result. - Fibonacci
The Fibonacci sequence is a series of integers (the Fibonacci numbers) like the following one:
0, 1, 1, 2, 3, 5, 8, 13, 21, 34
Every number after the first two (0 and 1) is the sum of the two preceding ones:
2 is the sum of the preceding two numbers – 1+1
0, 1, 1, 2, 3, 5, 8, 13, 21, 34
3 is the sum of the preceding two numbers – 1+2
And so on.
Task: Write a program that takes a positive integer N as an input and returns the N-th element of the Fibonacci sequence.
Hint: The easiest way to solve this is to use recursion, like in this example of Java code:
public int fib(int n){
if (n<2) return n;
return fib(n-1) + fib(n-2);
}
- Anagram
Anagrams are words with the same number of letters, but they may be arranged differently. For example, race and care, listen and silent.
Task: Write a program that receives two strings as an input and returns true if they are anagrams.
Hint: Sorting the two words and then comparing them is the quickest way, but it is not the best solution because usually sorting algorithms are time- and memory-consuming.
Another approach is to generate HashMaps of both words and then compare them. Use the HashMaps to check if they contain the same elements and if these elements occurred the same number of times in both maps.
Conclusion
Most people are anxious when going to an interview, especially if it is their first time.
Preparing in advance will always work in your favor giving you a leg up over the other candidates and the chance to make a good impression. Having an idea of the general process will help you come up with the best answers to the most common personal, technical, and practical questions when interviewing for a software engineer position.